How to URL Encode a String in Java Script
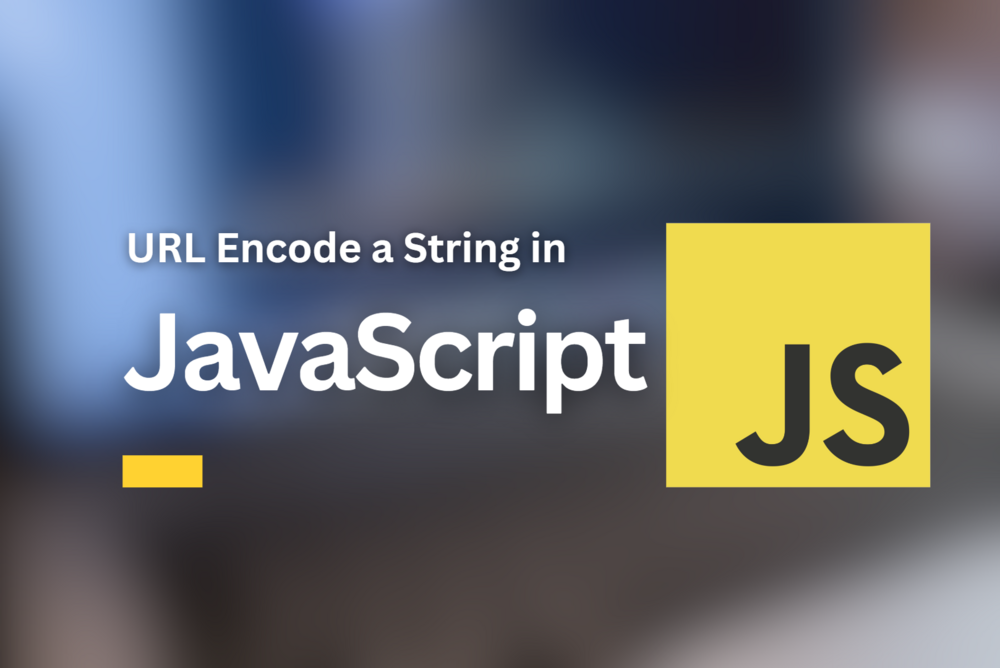
Introduction
URL encoding in JavaScript is a critical skill for web developers, as it allows you to safely include data in URLs, preventing errors and ensuring data integrity. In this guide, we'll explore how to URL encode a string in JavaScript and understand the underlying concepts.
URL Encoding in JavaScript
URL encoding is achieved using the encodeURIComponent()
function in JavaScript. This function takes a string as an argument and returns the string with all special and reserved characters replaced by their respective percent-encoded values.
Example Code:
// Original string with special characters const originalString = "Hello, World! This is a test."; // URL encoding the string const encodedString = encodeURIComponent(originalString); console.log("Original String:", originalString); console.log("Encoded String:", encodedString);
Explanation
-
We start with the
originalString
, which contains special characters, spaces, and punctuation. -
We use
encodeURIComponent(originalString)
to encode the string. This function replaces spaces with%20
, commas with%2C
, and other special characters with their respective percent-encoded values. -
Finally, we print both the original and encoded strings to the console for comparison.
Conclusion
URL encoding is a fundamental technique in web development to ensure data safety and integrity in URLs. By using encodeURIComponent()
in JavaScript, you can confidently handle data in your web applications, preventing errors and ensuring proper URL handling.