How to URL Encode a String in PHP
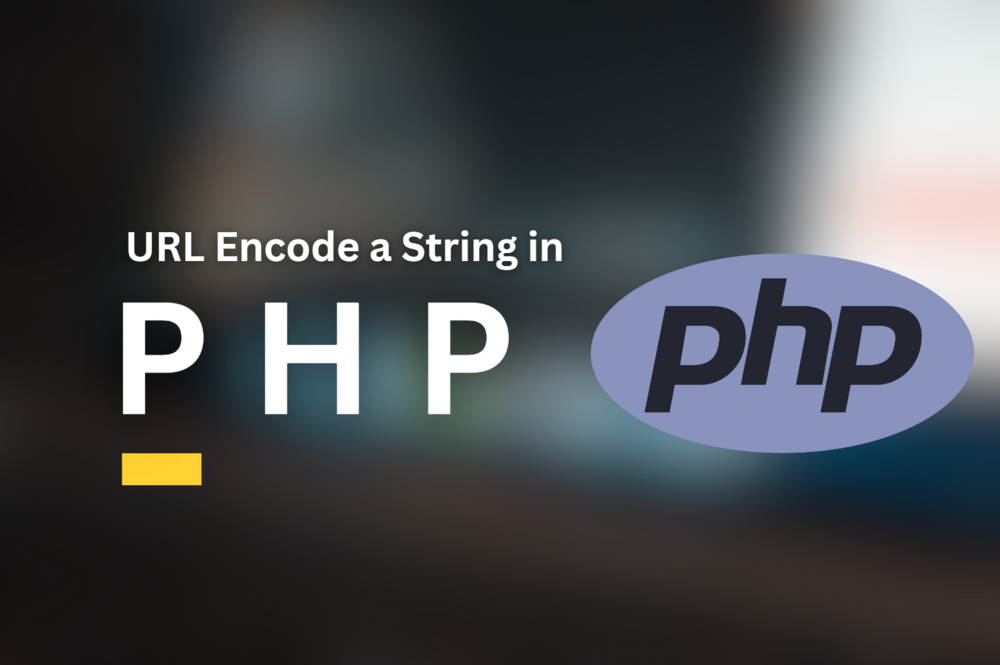
Introduction
URL encoding in PHP is a crucial process for ensuring that URLs can accurately represent various types of data, especially when dealing with special characters, spaces, and non-alphanumeric characters. It's an essential technique to maintain data integrity in web applications. In this guide, we will walk you through the process of URL encoding a string in PHP, providing both code examples and detailed explanations.
URL Encoding in PHP: Code and Explanation
PHP provides a built-in function called urlencode()
for URL encoding strings. Here's how you can use it:
<?php // Original string $originalString = "Hello World! How are you?"; // URL encode the string $encodedString = urlencode($originalString); // Display the encoded string echo "Encoded URL: " . $encodedString . "<br>"; ?>
Explanation
-
Original String: Define the original string that you want to encode. This can be any string containing spaces, special characters, or non-alphanumeric characters.
-
URL Encoding: Use the
urlencode()
function to encode the original string. The function replaces spaces with%20
and other reserved or special characters with their respective percent-encoded values. -
Display the Encoded String: Finally, you can display the encoded URL by echoing it. This encoded string can now be safely included in a URL.
Example Output
For the original string "Hello World! How are you?", the encoded URL would look like this:
#Output Encoded URL: Hello+World%21+How+are+you%3F
The spaces have been replaced with +
, the exclamation mark !
has been replaced with %21
, and the question mark ?
has been replaced with %3F
.
URL encoding ensures that the URL is properly formatted and that data is transmitted accurately, making it a crucial aspect of web development when dealing with user input and dynamic URLs.