How to URL Encode a String in Python
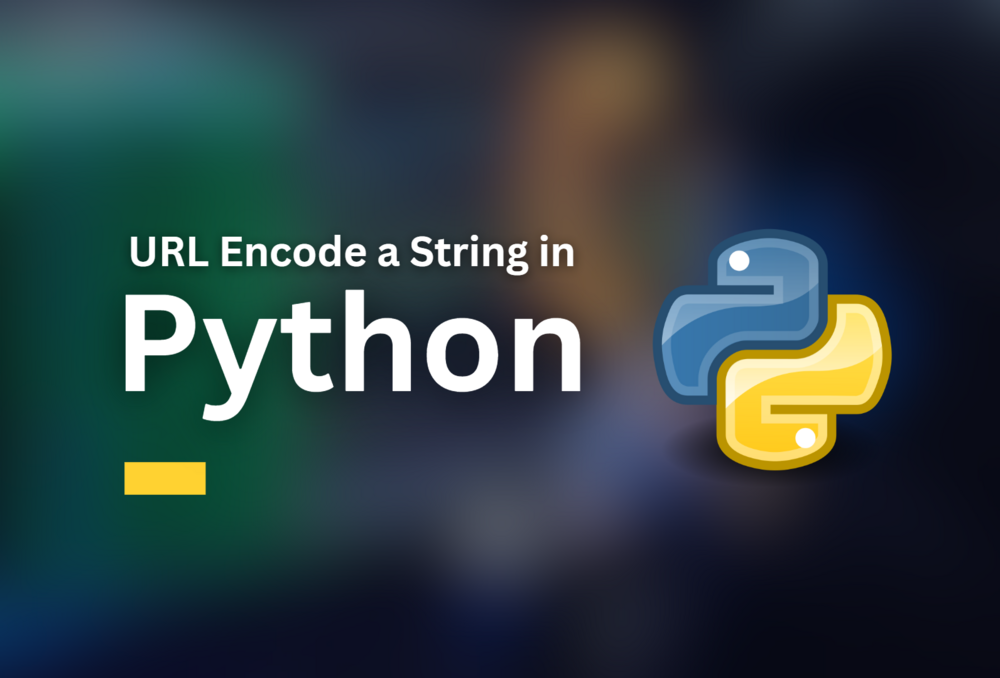
Introduction
URL encoding, also known as percent encoding, is a process used to convert special characters and spaces in a string into a format that can be safely included in a URL. In Python, the urllib.parse
module provides functions for URL encoding and decoding. This guide will show you how to URL encode a string in Python using the quote()
function from urllib.parse
.
Code and Explanation
Here's an example of how to URL encode a string in Python:
import urllib.parse # Original string with special characters original_string = "Hello, World! This is a test string with spaces and symbols like @ and #." # URL encode the string encoded_string = urllib.parse.quote(original_string) # Print the original and encoded strings print("Original String:", original_string) print("Encoded String:", encoded_string)
Explanation
-
Import the
urllib.parse
module to access the URL encoding functions. -
Define the
original_string
variable, which contains the string you want to URL encode. This string can include special characters, spaces, and symbols. -
Use the
urllib.parse.quote()
function to URL encode theoriginal_string
. This function takes care of replacing spaces and special characters with their percent-encoded representations. -
Finally, print both the original and encoded strings to see the transformation.
When you run this code, you'll notice that the encoded_string
now contains a URL-encoded version of the original_string
. Special characters like spaces, commas, and symbols have been replaced with percent-encoded values, making it safe for inclusion in a URL.
URL encoding is crucial when passing data in query parameters or constructing URLs dynamically in web development to ensure data integrity and compatibility with web standards.